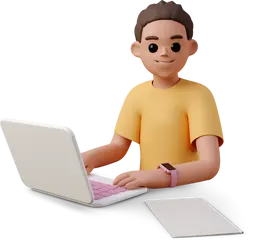
To automatically scroll a webpage with javascript
To automatically scroll a webpage with javascript, you can use the setInterval() function to repeatedly trigger a scrolling function.
(function (document) {
'use strict';
const ready = (callback) => {
if (document.readyState != 'loading') callback();
else document.addEventListener('DOMContentLoaded', callback);
};
ready(() => {
const target = document.body.scrollHeight - window.innerHeight;
const duration = 45000;
const distance = target / Math.floor(duration / 45);
const interval = setInterval(function () {
if (window.pageYOffset >= target) clearInterval(interval);
else window.scrollBy(0, distance);
}, 45);
});
})(document);