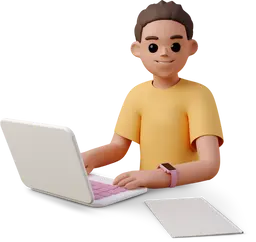
Tired of search inputs making unnecessary API calls? Learn how to debounce input fields in JavaScript
Introduction
Ever typed into a search bar and noticed that each keystroke triggers a new request? This can lead to unnecessary API calls, slow performance, and a bad user experience. The solution? Debouncing.
In this post, we'll build a DebouncedInput class, which delays function execution until the user stops typing for a specified time.
What is Debouncing?
Debouncing ensures that an event (like user input) only triggers after a short pause. Instead of firing a function every keystroke, we wait until the user stops typing.
🛑 Without Debounce → Calls function on every keypress.
✅ With Debounce → Calls function only after typing stops.
Creating the DebouncedInput
Class
Let’s build our reusable class:
class DebouncedInput {
constructor(selector, callback, delay = 300) {
this.inputElement = document.querySelector(selector);
if (!this.inputElement) return;
this.callback = callback;
this.delay = delay;
this.timeout = null;
this.handleInput = this.debounce(this.handleInput.bind(this), this.delay);
this.inputElement.addEventListener('input', this.handleInput);
}
debounce(func, wait) {
return (...args) => {
clearTimeout(this.timeout);
this.timeout = setTimeout(() => func.apply(this, args), wait);
};
}
handleInput(event) {
this.callback(event.target.value);
}
}
How It Works
- Constructor sets up the input field and debounce delay.
- Debounce function waits before executing the callback.
- handleInput() calls the provided function when typing stops.
Using DebouncedInput
Now, we can apply debounce to a search field:
const searchField = new DebouncedInput(
'#search',
(value) => {
console.log(`Fetching results for: ${value}`);
// Here you could make an API request or filter results
},
500
);
💡 Example: If the user types "apple"
, it waits 500ms after the last keystroke before executing console.log()
.
Benefits of Using Debounce
✅ Prevents excessive API calls – Only triggers when typing stops.
✅ Improves UX – Reduces lag and unnecessary requests.
✅ Optimized for performance – Ideal for live search suggestions.
Practical Use Cases
- 🔍 Search Bars – Only fetch results when the user stops typing.
- 📝 Form Validation – Check for valid input after the user finishes typing.
- 📊 Live Filtering – Update search results efficiently.
Conclusion
Debouncing is an essential technique for optimizing user input. With DebouncedInput
, you can easily debounce any text field without writing extra logic.
🚀 Try it in your projects and make your UI faster and more efficient! 🚀